In 2024, there were over 2 billion websites on the Internet, with more than 1.3 million using React to develop their frontend appearance and functionality for users. Today’s world of web development with React has made this tech one of the most popular tools for creating responsive and dynamic user experiences. If you are a beginner developer looking to get started with React, take a quick look at this MVP development services page by Celadonsoft or read this guide, which will help you get from the basics to more advanced concepts.
1. Overview
Meta originally created React as a library for constructing complicated website UI. Its based-on-components approach allows complicated interfaces to be broken down into simple, usable elements, simplifying application development and support.
2. The Configuration of Environment
Prior to deciding to work with RJS, you need to configure the development environment:
- The basis of installation: NJS enables JavaScript to execute outside the browser, whereas npm is used for package management. Download and install the most recent version of Node.js from the official site.
- Create a new project with the special command: Use the following code to initiate an app.
npx create-react-app the-app
cd my-app
npm start
- After this, the application will be available at the default host — http://localhost:3000/.
3. Component Fundamentals
React is structured around components. Due to this architecture, there are two types of them:
- Functional components are JavaScript functions that return JSX markup.
import React from 'react1';
const Welcome = () => {
return <h1>Hi Web Dev!</h1>;
- Class components: Although used less commonly with hooks, they are still significant.
import React, { Name_of_the_component} from 'react1';
class Welcome extends Name_of_the_component {
render() {
return <h1>Hi Web Dev!</h1>;
4. HTML-like Code
VirtualDOM is an important technique that enhances React’s capability and productivity. It allows React to effectively refresh the UI, decreasing the number of changes made to the actual DOM.
Also, JSX allows you to write HTML-style code inside JavaScript, making it more legible and declarative.
import React from 'react1';
…
const name = 'Polly';
return <h1>Hi, {name}!</h1>;
5. States and Props
State is a React mechanism that allows components to store and modify data. Unlike props, which are passed by the parent component and are immutable, state is controlled by the component itself and can be dynamically updated.
State:
import React, { useState } from 'react1';
…
const Calcucounter = () => {
const [count, setCount] = useState(0);
…
<p>Calculator: {count}</p>
<button onClick={() => setCount(count + 1)}>Expand</button>
…
Props:
import React from 'react1';
const Pleasantries = ({ name }) =>{
return <h1>Hi, {name}!</h1>;}
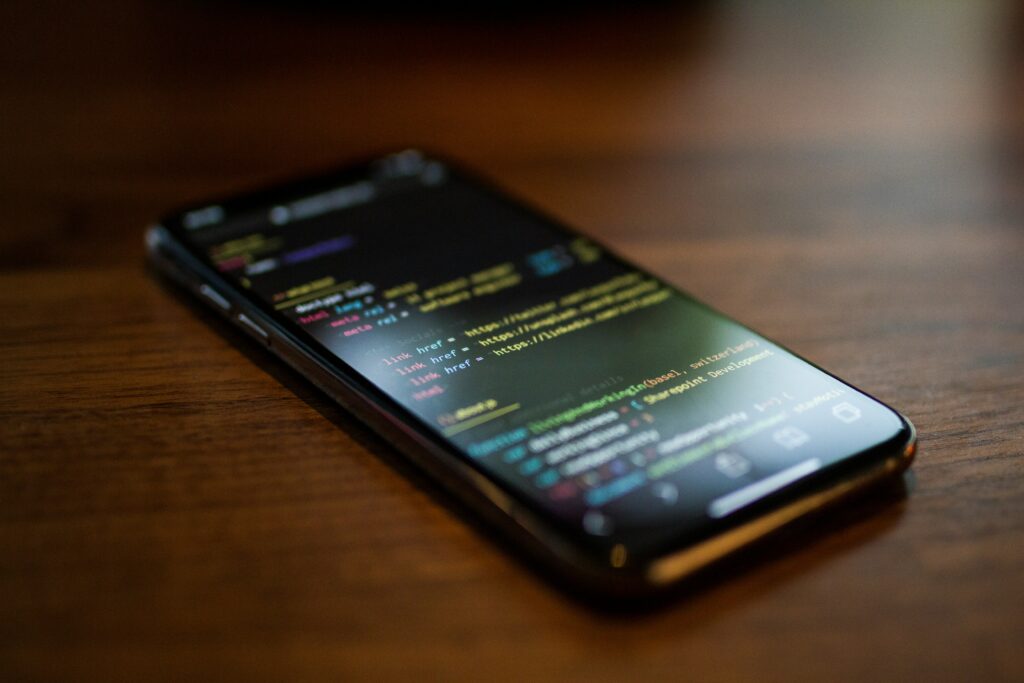
6. The Hooks Usage
Hooks let you use React’s state and other functionality in functional components.
Especially popular hooks:
- useState: for state management.
- useEffect: for side effects such as data loading.
- useContext: Share data between components without prop digging.
- useReducer: For sophisticated state logic.
- Custom-maded: Develop your own hooks for reusable logic.
7. Routeing by Router
React Router is used to create single-page applications with multiple views. It allows you to organize navigation within the application.
npm install react-router-dom
8. Component Styling
In web development with React, component structure options include CSS modules, which provide discrete styles for each component, and Styled Components, a JavaScript framework for authoring CSS using template literals.
9. Data Collecting from API
Modern applications often interact with external APIs to retrieve data. Use the useEffect hook to perform asynchronous queries.
import React, { useState, useEffect } from 'react1';
…
const DataFetcher = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
…
10. Components Testing
Testing ensures the app’s dependability and quality. The prominent tools in React are as follows:
- Jest is a testing framework for JavaScript programming.
- RTL is a collection of tools for testing React components.
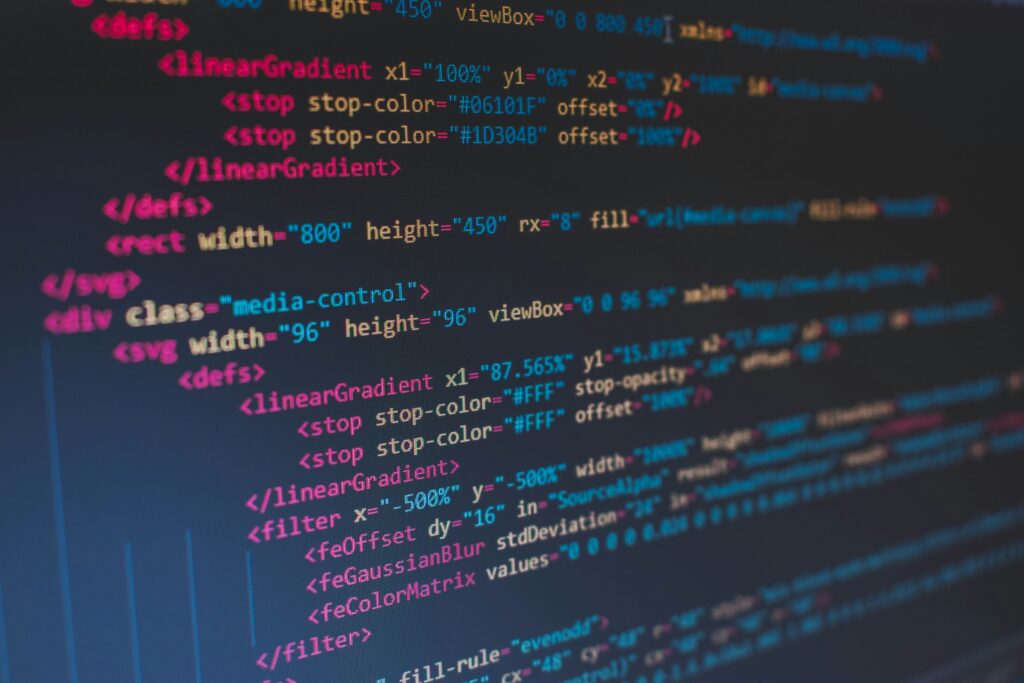
11. Deployment of the app
After the development is completed, the application should be deployed to users.
For the deployment of React-applications, you can use different platforms such as Netlify, Vercel and GitHub Pages. The easiest way is to deploy the application on Netlify.
This step will create an optimized version of the application in the build folder. Then upload it to Netlify or another hosting platform. Another option is to use Vercel, which allows you to deploy the application in just a few commands.
12. Single Page Applications (SPA) by React
“Single-page applications are web applications that load a single HTML document and dynamically update content without completely rebooting the page. This makes them fast, interactive and similar to native applications in the world of web development with React.” — Celadonsoft opinion.
Why SPA?
- Speed — the content is updated dynamically, without rebooting the entire page.
- Better user experience — smooth and instant page transitions.
- Flexibility — complex user interfaces and interactions can be easily implemented.
How Does React Help You Create a Spa?
React was originally created to build an SPA. Instead of loading a new HTML page with each request, React will render only the changed components. This is done by:
- React Router — to manage routes within the application.
- States (state) and props (props) — to manage data without rebooting.
- Component approach — for building overused UI elements.
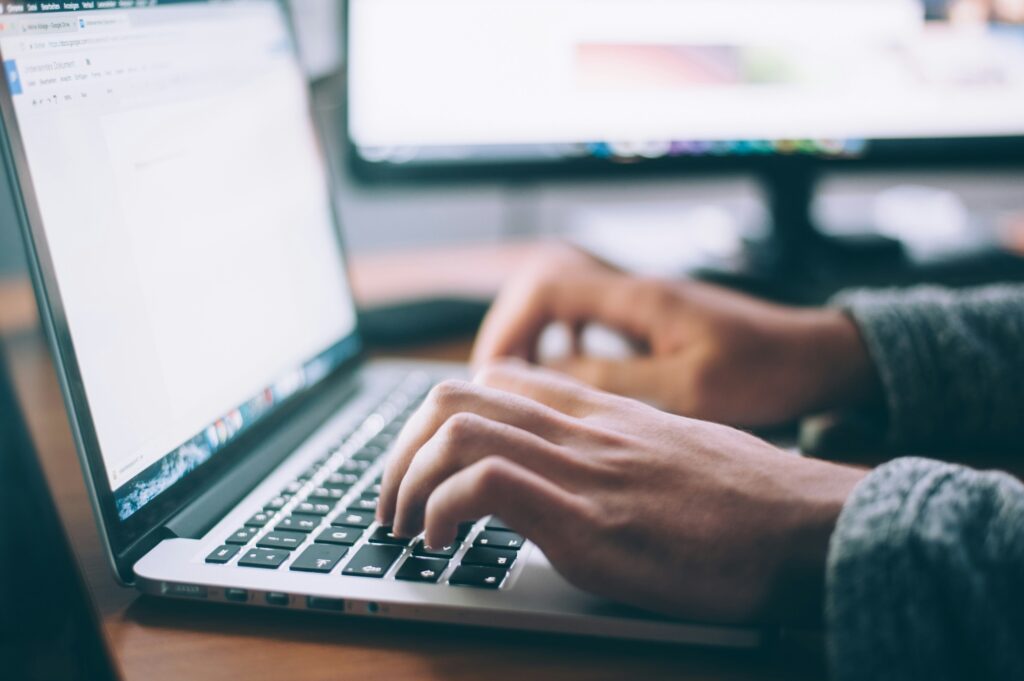
13. Future Horizons
Now you have a solid theory foundation to work with React. But the next steps can include learning:
- Redux — to manage the global state.
- Next.js — for server rendering and improved optimization.
- TypeScript — to improve the reliability of the code.
Summary
React is a great tool for building contemporary online apps. We’ve gone from the basics to more advanced concepts, including components, state, routing and working with APIs. Now you have a solid base for web development with React.
The most important thing is practice. Start with small applications, try to implement simple components, then move on to more complex tasks. Learn best practices, try new technologies such as Redux, Next.js and TypeScript.
The world of web development is evolving rapidly, and React remains at the center of this change.